Recently we have released our new image tool daycaca.
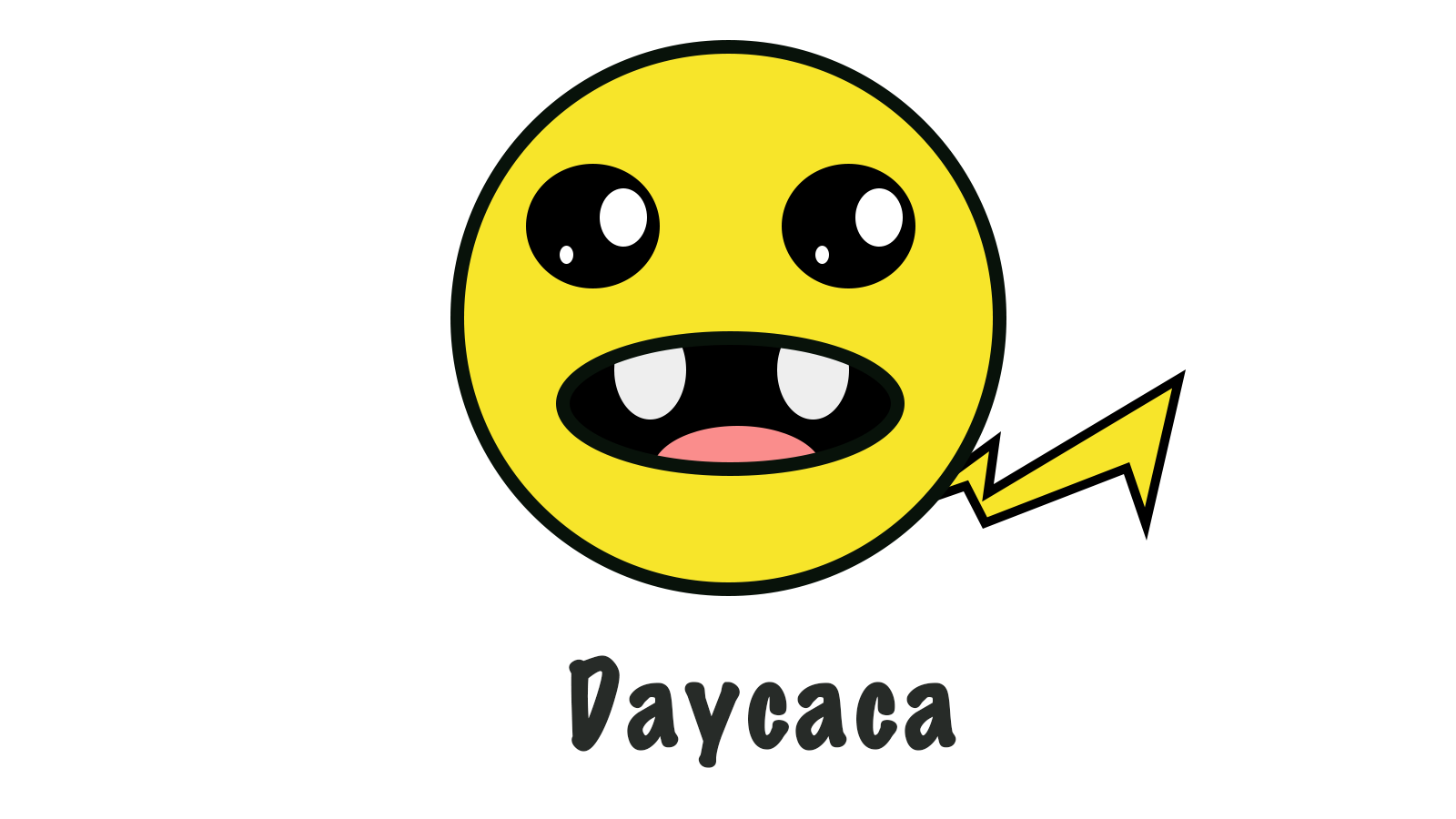
A pure JS library to handle image via canvas
<Canvas>
is a magic element which helps you to draw some amazing effects in a web page. And it enables a power to draw images on the canvas.
drawImage helps us draw an image on canvas. We can get each pixel data and change these data. toDataUrl method returns a data URI containing a representation of the image in the format specified by the type parameter. Those APIs leads to crop and compress image online.
How to use
Use Npm install the package.
$ npm install daycaca -save
// es6
import daycaca from 'daycaca';
// src specify an image src (url or base64)
daycaca.rotate(src, degress, (data, w, h) => {
// your code here
});
And you could import the script in your html file. And daycaca
attachs to the window
object.
<script src="./dist/daycaca.min.js"></script>
<script>
// src specify an image src (url or base64)
daycaca.rotate(src, degress, (data, w, h) => {
// your code here
});
</script>
API
All API methods's argument source
should be one type below:
- an image url (Pay attention to CORS settings)
- an IMG element
- a file object Which use
input[type="file"]
value as source
base64(source, callback)
Convert your image to base64.
const img = document.querySelector('img')
daycaca.base64(img, (data) => {
//... handle base64
})
compress(source, quailty, callback)
Compress your image and minify the size of your image.
-
PNG need lossless compression; So the param
quality
may not work. -
JPG/JPEG/BMP need lossy compression;
quality
(1~100). 100 means that the image keeps the same quality.
const img = document.querySelector('img')
daycaca.compress(img, 0.5,(data) => {
//... handle base64
})
crop(source, option, callback)
Crop your image to the size which you specify.
option {} :
- toCropImgX: The x-axis distance between the crop area and the image;
- toCropImgY: The y-axis distance between the crop area and the image;
- toCropImgW: The width of crop area;
- toCropImgH: The height of crop area
- ratio: the scale ration of the image
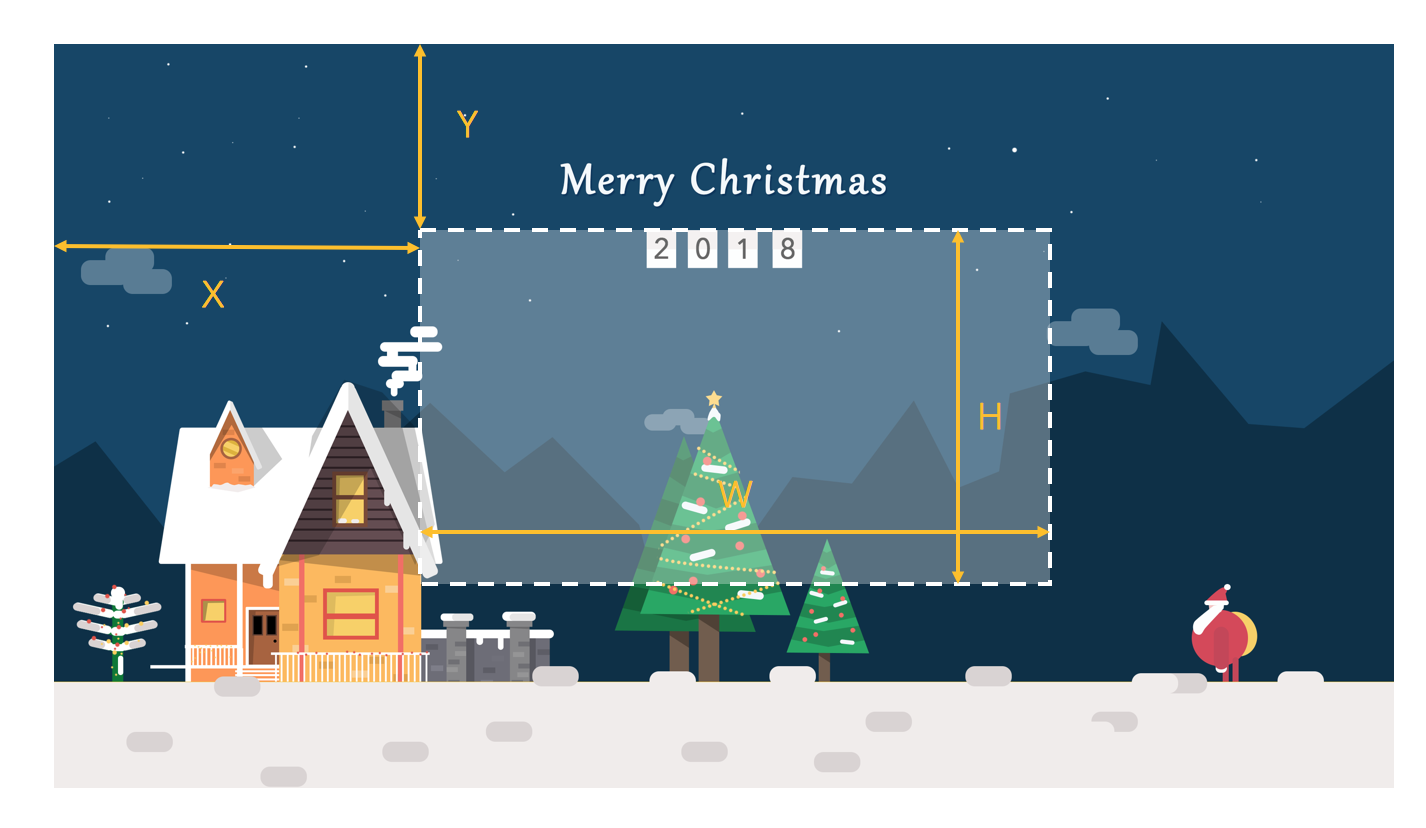
const img = document.querySelector('img')
daycaca.reszie(img, {
toCropImgX: 10,
toCropImgY: 20,
toCropImgW: 100,
toCropImgH: 70
},(data) => {
//... handle base64
})
rotate(source, degree, callback)
Rotate your image to any degree.
const img = document.querySelector('img')
daycaca.rotate(img, 90,(data) => {
//... handle base64
})
reszie(source, ratio, callback)
Scale the image;
- ratio (0~1): the scale ratio of the image. 1 means the image keep the same size;
const img = document.querySelector('img')
daycaca.reszie(img, 0.5,(data) => {
//... handle base64
})
Your contributions and suggestions are welcome 😄😄🌺🌺🎆🎆~